Finite State Machine: PongMaster
At the core of PongBot's functionality is the PongMaster node, which is a finite state machine that coordinates communication between navigation, acoustics, obstacle, and drive controller nodes.
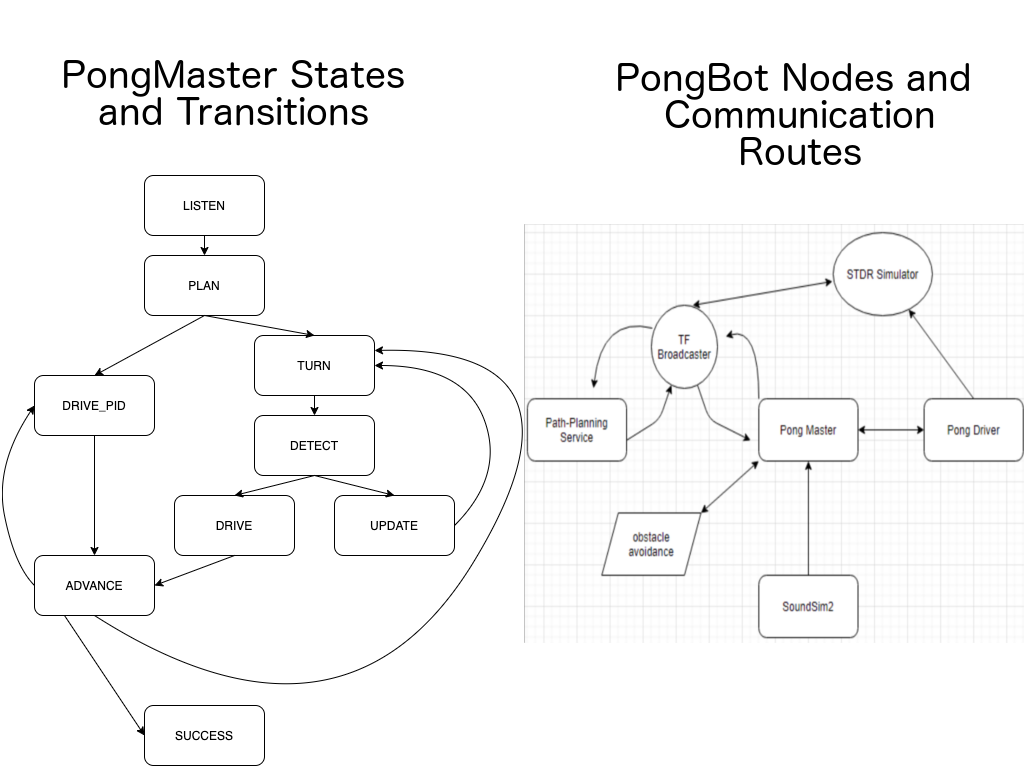
Our PongMaster has a number of states described below to coordinate robot functionality. These states transition to others, forming a loop that terminates upon achieving the goal pose.
Both Drive Routines
LISTEN: This is the first state our robot enters. It listens for our acoustics node to publish a target pose after the acoustics simulation has concluded. The LISTEN state transitions directly into our PLAN state.
PLAN: This state handles communication between PongMaster and our navigation node. It passes vital data such as our current and target poses to the navigation node, as well as the file path of our current map. If we are operating with our obstacle avoidance routine, we transition to the TURN state. If we are operating on pure PID, we transition to the DRIVE_PID state.
if Obstacle Avoidance Routine:
TURN: We only access this state when running our obstacle-avoidance routine. This state handles turning-on-a-dime until our robot is facing the next target pose. TURN exclusively transitions into the DETECT state.
DETECT: This state runs our obstacle detection algorithm, searching for obstacles directly in front of the robot. In the event that an obstacle is detected, we enter the UPDATE state.
UPDATE: We are in this state if an obstacle was detected in DETECT. This state handles updating our map with the location of the obstacle, as well as making a call to the navigation server to get the shortest possible route across the new map. Given a successful UPDATE, we transition back into the TURN state within the obstacle avoidance routine.
DRIVE: This state is entered if NO obstacle is detected in DETECT state, thus, signifying a clear path forward to the target pose. Once the target pose is achieved, we enter the ADVANCE state.
elif PID Drive Routine
DRIVE_PID: This state handles pure PID drive controls between our current and target locations. Upon achieving the target pose, we enter the ADVANCE state
Both Drive Routines
ADVANCE: This state is achieved whenever DRIVE or DRIVE_PID returns a successful response, signifying that we have achieved the current intermediate target pose. When entered, we signify to our navigation node that we need to update our target pose to the next location in our intermediate target array. Upon reaching the final goal pose, ADVANCE returns a SUCCESS flag to PongMaster, signifying goal completion. If we are not at our goal pose, we instead transition to the TURN or DRIVE_PID state, depending on which drive routine is selected.
SUCCESS: We did it! Success flag received by PongMaster.
Design Tradeoffs
Though very complex in implementation, we found that a state machine provided us with the flexibility necessary to test a number of different drive, navigation, and sound simulation nodes. We initially set out to have a greater number of pre-defined states for our robot to operate on. However, due to design complexity, many of these individual states were flattened into more complex states that handled a number of actions. Examples of this include the PLAN and ADVANCE states, which handle a number of different operations to provide us with the appropriate path to follow.
You may also notice that we neglected to include an error state within the above diagrams. Though there does exist a WAIT state in our code which actsa as an error state, we failed to implement routines to correct such errors and continue towards our goal pose.
FSM Analysis
When constructing this FSM, our group found much difficulty coordinating actions between different nodes that PongMaster. However, overcoming these integration issues yielded an efficient routine of actions that our robot could follow to reach a goal pose. By centralizing our FSM within a single node and serparating all other functionality to individual nodes, we were able to "hot-swap" node builds as they were developed in parallel.
Overall, we are extremely satisfied with the state of our PongMaster node. We believe that this FSM was the best, most efficient method of achieving our goals, while maintaining the ability to develop robot features individually. Future implementations of PongMaster would include a greater number of specialized states to increase code complexity and allow for further interchangeability. Lastly, we likely would leverage a developed ROS Finite State Machine package to simplify our implementation with existing frameworks.